Write a Program That Reads Integers From the User and Stores Them in a List. Reverse Ordeer
Are you diving deeper into Python lists and wanting to learn about different ways to reverse them? If so, then this tutorial is for you. Here, you'll learn virtually a few Python tools and techniques that are handy when it comes to reversing lists or manipulating them in contrary order. This knowledge will complement and improve your list-related skills and brand you more than proficient with them.
In this tutorial, you lot'll larn how to:
- Reverse existing lists in place using
.reverse()
and other techniques - Create reversed copies of existing lists using
reversed()
and slicing - Utilise iteration, comprehensions, and recursion to create reversed lists
- Iterate over your lists in reverse order
- Sort your lists in contrary club using
.sort()
andsorted()
To get the nigh out of this tutorial, it would be helpful to know the basics of iterables, for
loops, lists, list comprehensions, generator expressions, and recursion.
Reversing Python Lists
Sometimes you need to process Python lists starting from the terminal element down to the first—in other words, in opposite lodge. In full general, in that location are two main challenges related to working with lists in reverse:
- Reversing a list in place
- Creating reversed copies of an existing list
To meet the get-go claiming, yous tin use either .reverse()
or a loop that swaps items by index. For the second, you tin employ reversed()
or a slicing operation. In the adjacent sections, you'll larn about different ways to accomplish both in your code.
Reversing Lists in Place
Like other mutable sequence types, Python lists implement .reverse()
. This method reverses the underlying listing in identify for memory efficiency when y'all're reversing large list objects. Hither's how you can use .reverse()
:
>>>
>>> digits = [ 0 , 1 , ii , 3 , 4 , five , 6 , vii , 8 , 9 ] >>> digits . reverse () >>> digits [9, 8, 7, 6, 5, iv, 3, ii, i, 0]
When you call .reverse()
on an existing list, the method reverses information technology in identify. This way, when you access the list over again, you get it in contrary order. Note that .reverse()
doesn't return a new listing just None
:
>>>
>>> digits = [ 0 , 1 , ii , iii , 4 , v , vi , seven , eight , 9 ] >>> reversed_digits = digits . reverse () >>> reversed_digits is None True
Trying to assign the return value of .reverse()
to a variable is a common mistake related to using this method. The intent of returning None
is to remind its users that .opposite()
operates by side effect, changing the underlying list.
Okay! That was quick and straightforward! Now, how tin you opposite a list in identify past hand? A common technique is to loop through the first one-half of it while swapping each element with its mirror counterpart on the 2d half of the listing.
Python provides zero-based positive indices to walk sequences from left to correct. It also allows y'all to navigate sequences from right to left using negative indices:
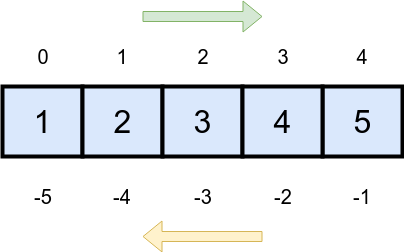
This diagram shows that you can access the first element of the list (or sequence) using either 0
or -5
with the indexing operator, like in sequence[0]
and sequence[-5]
, respectively. You can use this Python feature to opposite the underlying sequence in place.
For case, to reverse the list represented in the diagram, you can loop over the first half of the list and bandy the element at alphabetize 0
with its mirror at index -1
in the commencement iteration. Then y'all can switch the element at index 1
with its mirror at index -ii
and then on until y'all get the list reversed.
Here's a representation of the whole procedure:
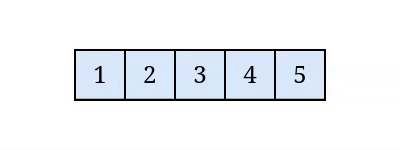
To interpret this process into code, you lot tin can employ a for
loop with a range
object over the get-go one-half of the list, which you tin go with len(digits) // 2
. Then you lot can use a parallel assignment argument to swap the elements, similar this:
>>>
>>> digits = [ 0 , i , 2 , 3 , 4 , v , 6 , vii , 8 , nine ] >>> for i in range ( len ( digits ) // two ): ... digits [ i ], digits [ - 1 - i ] = digits [ - ane - i ], digits [ i ] ... >>> digits [nine, 8, 7, 6, 5, iv, 3, ii, 1, 0]
This loop iterates through a range
object that goes from 0
to len(digits) // 2
. Each iteration swaps an particular from the first half of the listing with its mirror counterpart in the second half. The expression -i - i
inside the indexing operator, []
, guarantees access to the mirror item. Y'all can also use the expression -1 * (i + i)
to provide the corresponding mirror index.
Besides the above algorithm, which takes reward of index substitution, there are a few different means to reverse lists by hand. For case, you can use .pop()
and .insert()
like this:
>>>
>>> digits = [ 0 , 1 , 2 , 3 , 4 , five , half-dozen , seven , 8 , 9 ] >>> for i in range ( len ( digits )): ... last_item = digits . pop () ... digits . insert ( i , last_item ) ... >>> digits [9, 8, seven, half dozen, v, four, iii, 2, 1, 0]
In the loop, yous call .pop()
on the original list without arguments. This call removes and returns the terminal item in the list, so you lot can store it in last_item
. And so .insert()
moves last_item
to the position at index i
.
For example, the first iteration removes 9
from the right end of the list and stores it in last_item
. Then it inserts 9
at index 0
. The adjacent iteration takes eight
and moves it to index 1
, and so on. At the end of the loop, you lot become the list reversed in place.
Creating Reversed Lists
If y'all desire to create a reversed re-create of an existing list in Python, and then you can use reversed()
. With a listing every bit an argument, reversed()
returns an iterator that yields items in reverse order:
>>>
>>> digits = [ 0 , 1 , two , 3 , iv , five , 6 , 7 , 8 , 9 ] >>> reversed_digits = reversed ( digits ) >>> reversed_digits <list_reverseiterator object at 0x7fca9999e790> >>> list ( reversed_digits ) [ix, eight, 7, 6, 5, 4, 3, ii, 1, 0]
In this case, you call reversed()
with digits
as an argument. So you store the resulting iterator in reversed_digits
. The call to list()
consumes the iterator and returns a new list containing the same items as digits
but in reverse order.
An of import point to annotation when you're using reversed()
is that information technology doesn't create a copy of the input list, so changes on it affect the resulting iterator:
>>>
>>> fruits = [ "apple" , "assistant" , "orangish" ] >>> reversed_fruit = reversed ( fruits ) # Get the iterator >>> fruits [ - 1 ] = "kiwi" # Alter the last item >>> adjacent ( reversed_fruit ) # The iterator sees the modify 'kiwi'
In this case, you call reversed()
to go the corresponding iterator over the items in fruits
. And so yous modify the last fruit. This change affects the iterator. You tin ostend that by calling next()
to get the start item in reversed_fruit
.
If you need to go a copy of fruits
using reversed()
, and so you can call list()
:
>>>
>>> fruits = [ "apple" , "assistant" , "orange" ] >>> list ( reversed ( fruits )) ['orangish', 'banana', 'apple']
Equally you already know, the phone call to list()
consumes the iterator that results from calling reversed()
. This way, y'all create a new list every bit a reversed copy of the original one.
Python 2.4 added reversed()
, a universal tool to facilitate reverse iteration over sequences, as stated in PEP 322. In general, reversed()
tin accept whatsoever objects that implement a .__reversed__()
method or that support the sequence protocol, consisting of the .__len__()
and .__getitem__()
special methods. And then, reversed()
isn't limited to lists:
>>>
>>> list ( reversed ( range ( 10 ))) [9, viii, 7, half dozen, five, 4, three, 2, 1, 0] >>> listing ( reversed ( "Python" )) ['n', 'o', 'h', 't', 'y', 'P']
Here, instead of a list, you pass a range
object and a string equally arguments to reversed()
. The function does its job as expected, and you go a reversed version of the input data.
Another important point to highlight is that you lot can't use reversed()
with capricious iterators:
>>>
>>> digits = iter ([ 0 , i , ii , 3 , iv , 5 , 6 , 7 , 8 , nine ]) >>> reversed ( digits ) Traceback (virtually recent phone call terminal): File "<stdin>", line 1, in <module> TypeError: 'list_iterator' object is not reversible
In this case, iter()
builds an iterator over your list of numbers. When you telephone call reversed()
on digits
, you get a TypeError
.
Iterators implement the .__next__()
special method to walk through the underlying information. They're likewise expected to implement the .__iter__()
special method to return the current iterator instance. However, they're not expected to implement either .__reversed__()
or the sequence protocol. So, reversed()
doesn't work for them. If you ever need to reverse an iterator similar this, then you should beginning convert it to a list using list()
.
Another point to notation is that you can't use reversed()
with unordered iterables:
>>>
>>> digits = { 0 , 1 , ii , 3 , 4 , five , six , 7 , eight , nine } >>> reversed ( digits ) Traceback (nearly recent call last): File "<stdin>", line 1, in <module> TypeError: 'set' object is not reversible
In this example, when you try to utilize reversed()
with a set
object, you get a TypeError
. This is because sets don't proceed their items ordered, so Python doesn't know how to reverse them.
Reversing Lists Through Slicing
Since Python i.4, the slicing syntax has had a third argument, chosen step
. However, that syntax initially didn't piece of work on built-in types, such as lists, tuples, and strings. Python 2.3 extended the syntax to built-in types, then yous can apply footstep
with them now. Here'due south the full-blown slicing syntax:
This syntax allows yous to extract all the items in a_list
from start
to end − 1
past footstep
. The third offset, step
, defaults to 1
, which is why a normal slicing functioning extracts the items from left to right:
>>>
>>> digits = [ 0 , 1 , ii , three , 4 , 5 , 6 , 7 , 8 , 9 ] >>> digits [ 1 : 5 ] [one, two, three, iv]
With [1:5]
, yous get the items from index 1
to index five - 1
. The detail with the index equal to cease
is never included in the last result. This slicing returns all the items in the target range because step
defaults to one
.
If y'all utilize a unlike step
, then the slicing jumps every bit many items as the value of step
:
>>>
>>> digits = [ 0 , 1 , two , iii , 4 , 5 , 6 , 7 , viii , nine ] >>> digits [ 0 :: 2 ] [0, 2, 4, vi, 8] >>> digits [:: 3 ] [0, 3, vi, ix]
In the first example, [0::2]
extracts all items from index 0
to the end of digits
, jumping over two items each fourth dimension. In the second example, the slicing jumps 3
items as it goes. If y'all don't provide values to start
and stop
, so they are set to 0
and to the length of the target sequence, respectively.
If you set up stride
to -ane
, then you get a slice with the items in contrary order:
>>>
>>> digits = [ 0 , 1 , 2 , iii , 4 , five , half dozen , vii , 8 , 9 ] >>> # Ready pace to -1 >>> digits [ len ( digits ) - 1 :: - 1 ] [9, eight, vii, 6, v, four, three, 2, ane, 0] >>> digits [0, one, 2, three, 4, 5, half-dozen, 7, 8, nine]
This slicing returns all the items from the correct end of the listing (len(digits) - 1
) back to the left end because y'all omit the second offset. The balance of the magic in this instance comes from using a value of -1
for footstep
. When you lot run this trick, you get a copy of the original list in contrary order without affecting the input data.
If y'all fully rely on implicit offsets, so the slicing syntax gets shorter, cleaner, and less fault-prone:
>>>
>>> digits = [ 0 , i , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 ] >>> # Rely on default starting time values >>> digits [:: - 1 ] [9, 8, 7, 6, five, 4, three, 2, 1, 0]
Here, you ask Python to give you the complete list ([::-1]
) but going over all the items from back to front by setting step
to -1
. This is pretty neat, simply reversed()
is more efficient in terms of execution time and memory usage. It'south also more readable and explicit. Then these are points to consider in your code.
Another technique to create a reversed copy of an existing list is to utilize piece()
. The signature of this born part is like this:
This function works similarly to the indexing operator. It takes three arguments with similar meaning to those used in the slicing operator and returns a slice object representing the fix of indices returned by range(showtime, terminate, pace)
. That sounds complicated, so hither are some examples of how slice()
works:
>>>
>>> digits = [ 0 , one , two , iii , four , v , 6 , 7 , 8 , 9 ] >>> piece ( 0 , len ( digits )) slice(0, x, None) >>> digits [ piece ( 0 , len ( digits ))] [0, 1, ii, 3, iv, 5, 6, 7, 8, 9] >>> slice ( len ( digits ) - 1 , None , - one ) piece(9, None, -1) >>> digits [ slice ( len ( digits ) - 1 , None , - i )] [9, 8, vii, six, v, 4, iii, 2, 1, 0]
The commencement call to slice()
is equivalent to [0:len(digits)]
. The 2d call works the same as [len(digits) - i::-1]
. Y'all can also emulate the slicing [::-1]
using slice(None, None, -1)
. In this case, passing None
to commencement
and cease
means that you want a piece from the get-go to the end of the target sequence.
Hither's how you tin use slice()
to create a reversed copy of an existing list:
>>>
>>> digits = [ 0 , ane , ii , 3 , 4 , five , half dozen , 7 , 8 , 9 ] >>> digits [ slice ( None , None , - i )] [9, 8, vii, half dozen, 5, 4, 3, 2, 1, 0]
The slice
object extracts all the items from digits
, starting from the right end back to the left terminate, and returns a reversed copy of the target list.
Generating Reversed Lists by Hand
Then far, you've seen a few tools and techniques to either reverse lists in identify or create reversed copies of existing lists. Near of the fourth dimension, these tools and techniques are the way to go when it comes to reversing lists in Python. However, if you ever demand to reverse lists past hand, and so it'd be beneficial for you to understand the logic behind the process.
In this section, you'll acquire how to reverse Python lists using loops, recursion, and comprehensions. The thought is to get a listing and create a copy of it in contrary club.
Using a Loop
The first technique y'all'll use to reverse a list involves a for
loop and a list concatenation using the plus symbol (+
):
>>>
>>> digits = [ 0 , 1 , two , 3 , iv , v , six , vii , 8 , nine ] >>> def reversed_list ( a_list ): ... event = [] ... for item in a_list : ... result = [ item ] + issue ... return result ... >>> reversed_list ( digits ) [nine, viii, 7, vi, 5, 4, 3, 2, 1, 0]
Every iteration of the for
loop takes a subsequent particular from a_list
and creates a new list that results from concatenating [particular]
and result
, which initially holds an empty listing. The newly created list is reassigned to result
. This role doesn't change a_list
.
You tin can also have advantage of .insert()
to create reversed lists with the help of a loop:
>>>
>>> digits = [ 0 , i , 2 , 3 , 4 , v , vi , 7 , 8 , 9 ] >>> def reversed_list ( a_list ): ... effect = [] ... for item in a_list : ... result . insert ( 0 , detail ) ... return outcome ... >>> reversed_list ( digits ) [9, viii, 7, half-dozen, 5, four, 3, 2, one, 0]
The call to .insert()
within the loop inserts subsequent items at the 0
index of result
. At the end of the loop, you go a new list with the items of a_list
in reverse social club.
Using .insert()
similar in the to a higher place example has a pregnant drawback. Insert operations at the left finish of Python lists are known to be inefficient regarding execution fourth dimension. That's because Python needs to move all the items one step back to insert the new detail at the first position.
Using Recursion
You can also use recursion to reverse your lists. Recursion is when you ascertain a function that calls itself. This creates a loop that can become infinite if you don't provide a base of operations case that produces a result without calling the function once again.
Yous need the base case to end the recursive loop. When information technology comes to reversing lists, the base example would be reached when the recursive calls get to the end of the input list. You as well need to define the recursive case, which reduces all successive cases toward the base case and, therefore, to the loop'southward cease.
Here'due south how yous tin define a recursive function to return a reversed re-create of a given listing:
>>>
>>> digits = [ 0 , ane , 2 , 3 , 4 , five , vi , seven , 8 , nine ] >>> def reversed_list ( a_list ): ... if len ( a_list ) == 0 : # Base of operations case ... render a_list ... else : ... # print(a_list) ... # Recursive case ... return reversed_list ( a_list [ 1 :]) + a_list [: 1 ] ... >>> reversed_list ( digits ) [nine, 8, vii, six, v, 4, iii, 2, 1, 0]
Within reversed_list()
, yous commencement check the base of operations case, in which the input list is empty and makes the office return. The else
clause provides the recursive case, which is a call to reversed_list()
itself but with a slice of the original list, a_list[1:]
. This slice contains all the items in a_list
except for the showtime detail, which is then added as a single-item list (a_list[:one]
) to the outcome of the recursive call.
The commented phone call to print()
at the beginning of the else
clause is just a play tricks intended to show how subsequent calls reduce the input list toward the base case. Become alee and uncomment the line to meet what happens!
Using a List Comprehension
If yous're working with lists in Python, then you probably desire to consider using a list comprehension. This tool is quite popular in the Python space considering it represents the Pythonic mode to process lists.
Here's an example of how to utilise a list comprehension to create a reversed list:
>>>
>>> digits = [ 0 , 1 , 2 , 3 , 4 , 5 , 6 , vii , 8 , 9 ] >>> last_index = len ( digits ) - 1 >>> [ digits [ i ] for i in range ( last_index , - 1 , - i )] [ix, eight, 7, six, 5, 4, iii, 2, i, 0]
The magic in this list comprehension comes from the call to range()
. In this case, range()
returns indices from len(digits) - i
dorsum to 0
. This makes the comprehension loop iterate over the items in digits
in reverse, creating a new reversed list in the procedure.
Iterating Through Lists in Reverse
Upwardly to this indicate, you've learned how to create reversed lists and also how to reverse existing lists in place, either by using tools peculiarly designed to accomplish that task or by using your ain hand-coded solutions.
In 24-hour interval-to-day programming, you might find that iterating through existing lists and sequences in reverse club, typically known as contrary iteration, is a fairly mutual requirement. If that's your case, and so you take several options. Depending on your specific needs, you lot tin use:
- The built-in role
reversed()
- The slicing operator,
[::]
- The special method
.__reversed__()
In the following few sections, you'll acquire about all these options and how they tin assistance you iterate over lists in contrary order.
The Born reversed()
Role
Your first approach to iterating over a list in reverse order might be to utilise reversed()
. This born office was specially designed to support contrary iteration. With a listing as an statement, it returns an iterator that yields the input listing items in opposite social club.
Here's how you tin utilise reversed()
to iterate through the items in a list in opposite order:
>>>
>>> digits = [ 0 , one , two , 3 , 4 , 5 , vi , 7 , 8 , nine ] >>> for digit in reversed ( digits ): ... print ( digit ) ... 9 viii vii 6 5 4 three 2 1 0
The first matter to note in this example is that the for
loop is highly readable. The name of reversed()
clearly expresses its intent, with the subtle detail of communicating that the part doesn't produce any side effects. In other words, it doesn't alter the input list.
The loop is as well efficient in terms of memory usage because reversed()
returns an iterator that yields items on demand without storing them all in memory at the aforementioned fourth dimension. Again, a subtle detail to note is that if the input listing changes during the iteration, so the iterator sees the changes.
The Slicing Operator, [::-1]
The second approach to reverse iteration is to employ the extended slicing syntax you saw before. This syntax does nil in favor of memory efficiency, beauty, or clarity. Still, information technology provides a quick way to iterate over a reversed re-create of an existing list without the hazard of being afflicted by changes in the original listing.
Here's how you can use [::-one]
to iterate through a copy of an existing list in reverse social club:
>>>
>>> digits = [ 0 , 1 , ii , 3 , 4 , 5 , 6 , seven , eight , 9 ] >>> for digit in digits [:: - 1 ]: ... impress ( digit ) ... 9 8 7 6 five 4 three 2 1 0
When you slice a list similar in this example, you create a reversed re-create of the original list. Initially, both lists contain references to the same group of items. However, if yous assign a new value to a given item in the original list, like in digits[0] = "zero"
, then the reference changes to point to the new value. This mode, changes on the input list don't affect the re-create.
You lot can accept reward of this kind of slicing to safely modify the original listing while yous iterate over its old items in reverse order. For instance, say you need to iterate over a list of numbers in opposite order and supercede every number with its square value. In this case, yous can do something like this:
>>>
>>> numbers = [ 0 , 1 , two , 3 , iv , 5 , half dozen , vii , viii , 9 ] >>> for i , number in enumerate ( numbers [:: - 1 ]): ... numbers [ i ] = number ** 2 ... >>> # Foursquare values in opposite order >>> numbers [81, 64, 49, 36, 25, 16, 9, 4, 1, 0]
Hither, the loop iterates through a reversed copy of numbers
. The call to enumerate()
provides ascending zero-based indices for each particular in the reversed re-create. That allows you to modify numbers
during the iteration. Then the loop modifies the numbers
by replacing each item with its square value. As a result, numbers
ends upwardly containing square values in reverse order.
The Special Method .__reversed__()
Python lists implement a special method called .__reversed__()
that enables reverse iteration. This method provides the logic behind reversed()
. In other words, a telephone call to reversed()
with a list equally an argument triggers an implicit telephone call to .__reversed__()
on the input list.
This special method returns an iterator over the items of the current listing in reverse lodge. However, .__reversed__()
isn't intended to exist used straight. Most of the time, you'll use it to equip your own classes with reverse iteration capabilities.
For instance, say y'all want to iterate over a range of floating-point numbers. You can't use range()
, so you decide to create your ain grade to approach this specific utilize case. You cease upwardly with a class like this:
# float_range.py form FloatRange : def __init__ ( self , kickoff , end , footstep = 1.0 ): if start >= stop : raise ValueError ( "Invalid range" ) self . start = start cocky . stop = terminate self . step = pace def __iter__ ( self ): north = self . kickoff while n < cocky . stop : yield n n += self . step def __reversed__ ( self ): northward = self . stop - self . step while n >= self . starting time : yield n northward -= self . step
This class isn't perfect. It's simply your first version. However, it allows yous to iterate through an interval of floating-point numbers using a fixed increment value, stride
. In your form, .__iter__()
provides back up for normal iteration and .__reversed__()
supports reverse iteration.
To employ FloatRange
, you can exercise something similar this:
>>>
>>> from float_range import FloatRange >>> for number in FloatRange ( 0.0 , 5.0 , 0.5 ): ... print ( number ) ... 0.0 0.5 1.0 1.five 2.0 2.v 3.0 3.5 iv.0 4.5
The class supports normal iteration, which, as mentioned, is provided past .__iter__()
. Now you can attempt to iterate in reverse order using reversed()
:
>>>
>>> from float_range import FloatRange >>> for number in reversed ( FloatRange ( 0.0 , 5.0 , 0.v )): ... print ( number ) ... 4.5 iv.0 3.5 3.0 2.5 2.0 1.v ane.0 0.5 0.0
In this example, reversed()
relies on your .__reversed__()
implementation to provide the reverse iteration functionality. This way, you have a working floating-point iterator.
Reversing Python Lists: A Summary
Up to this bespeak, y'all've learned a lot about reversing lists using unlike tools and techniques. Here's a table that summarizes the more important points you've already covered:
Characteristic | .reverse() | reversed() | [::-one] | Loop | List Comp | Recursion |
---|---|---|---|---|---|---|
Modifies the list in place | ✔ | ❌ | ❌ | ✔/❌ | ❌ | ❌ |
Creates a copy of the list | ❌ | ❌ | ✔ | ✔/❌ | ✔ | ✔ |
Is fast | ✔ | ✔ | ❌ | ❌ | ✔ | ❌ |
Is universal | ❌ | ✔ | ✔ | ✔ | ✔ | ✔ |
A quick look at this summary volition permit you lot to decide which tool or technique to use when yous're reversing lists in place, creating reversed copies of existing lists, or iterating over your lists in reverse guild.
Sorting Python Lists in Reverse Gild
Another interesting selection when it comes to reversing lists in Python is to use .sort()
and sorted()
to sort them in contrary order. To do that, you can laissez passer True
to their respective reverse
argument.
The goal of .sort()
is to sort the items of a list. The sorting is done in place, so it doesn't create a new listing. If you set the contrary
keyword statement to True
, and then you get the list sorted in descending or reverse order:
>>>
>>> digits = [ 0 , v , seven , iii , 4 , ix , 1 , 6 , 3 , 8 ] >>> digits . sort ( opposite = Truthful ) >>> digits [9, eight, seven, 6, 5, 4, iii, 3, 1, 0]
Now your listing is fully sorted and besides in reverse order. This is quite user-friendly when you're working with some data and you need to sort it and contrary information technology at the same time.
On the other hand, if you want to iterate over a sorted list in reverse order, then you lot tin utilize sorted()
. This built-in function returns a new list containing all the items of the input iterable in order. If you pass Truthful
to its opposite
keyword argument, then you get a reversed copy of the initial list:
>>>
>>> digits = [ 0 , 5 , vii , iii , 4 , nine , 1 , vi , iii , 8 ] >>> sorted ( digits , contrary = True ) [9, 8, vii, 6, 5, four, 3, 3, 1, 0] >>> for digit in sorted ( digits , reverse = True ): ... print ( digit ) ... ix 8 7 6 5 four 3 3 1 0
The reverse
argument to sorted()
allows you to sort iterables in descending society instead of in ascending order. So, if y'all need to create sorted lists in reverse order, then sorted()
is for you.
Conclusion
Reversing and working with lists in reverse order might be a fairly common task in your twenty-four hours-to-day work every bit a Python coder. In this tutorial, you took advantage of a couple of Python tools and techniques to reverse your lists and manage them in reverse club.
In this tutorial, you learned how to:
- Reverse your lists in place using
.contrary()
and other techniques - Utilise
reversed()
and slicing to create reversed copies of your lists - Use iteration, comprehensions, and recursion to create reversed lists
- Iterate through your lists in opposite society
- Sort lists in reverse gild using
.sort()
andsorted()
All of this knowledge helps you improve your list-related skills. Information technology provides you with the required tools to be more than skillful when you're working with Python lists.
giddingsdigetund66.blogspot.com
Source: https://realpython.com/python-reverse-list/
Belum ada Komentar untuk "Write a Program That Reads Integers From the User and Stores Them in a List. Reverse Ordeer"
Posting Komentar